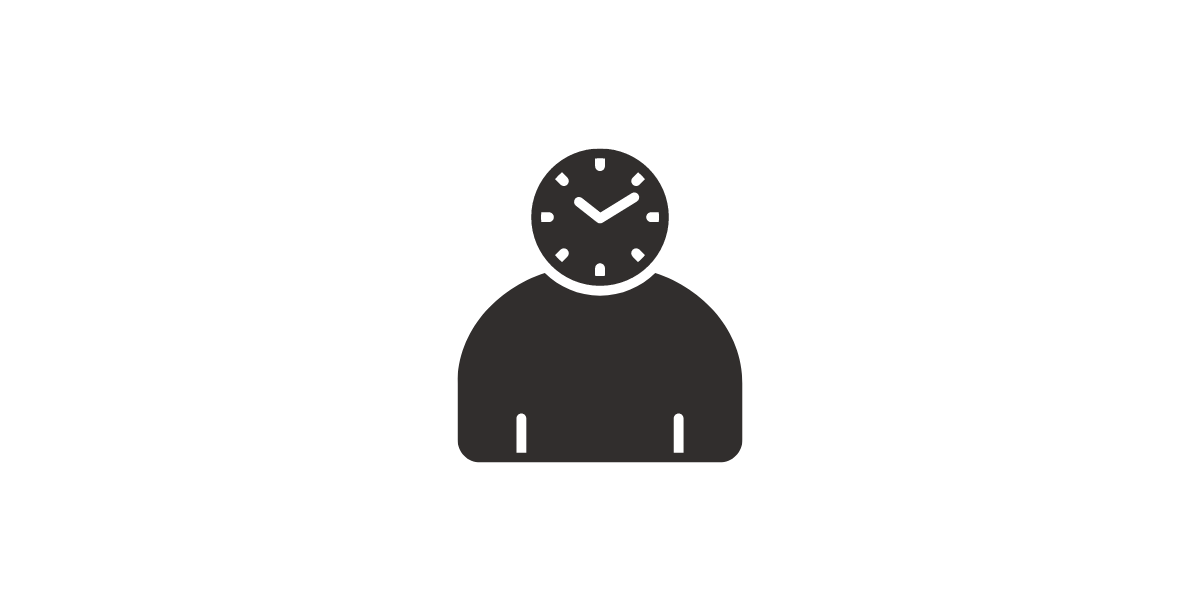
All variables belong to a scope
Let's create a variable a
in the main scope and variable b
in a local scope.
We'll then assign variable a
to b
and add a print statement in the main scope.
This program will not compile. That is because variable a
is referencing a variable b
that does not live outside its scope.
b
's lifetime only lasts within a temporary scope. This is Rust's mechanism for avoiding dangling pointers and the reason it is considered a safe language.
A longer-lasting variable
What if we want a variable to last longer than its existing scope.
Here is where lifetimes come into play. Lifetimes allow a reference to a variable to remain valid as long as needed.
In the code snippet below, the program does not compile.
The reason being as before, variable x
is making use of a short-lived variable y
.
Let's introduce lifetimes to the code to make it compile.
Here we've declared 2 lifetimes a and b using angle brackets. We've asked the compiler to allow a lifetime a to the returned value of the function func
.
Lifetime a is equivalent to the lifetime of variable z
found in the main scope. The program now compiles successfully.
If we instead give the return value of func
lifetime b, the program will not compile because lifetime b is equivalent to the local scope where y
lives.
Summary:
- Variables created within a scope
{}
live as long as that scope lasts. - A reference to a variable is allowed so long as that variable exists. Rust's way of avoiding dangling pointers.
- Lifetimes allow a reference to a variable to remain valid for as long as needed.